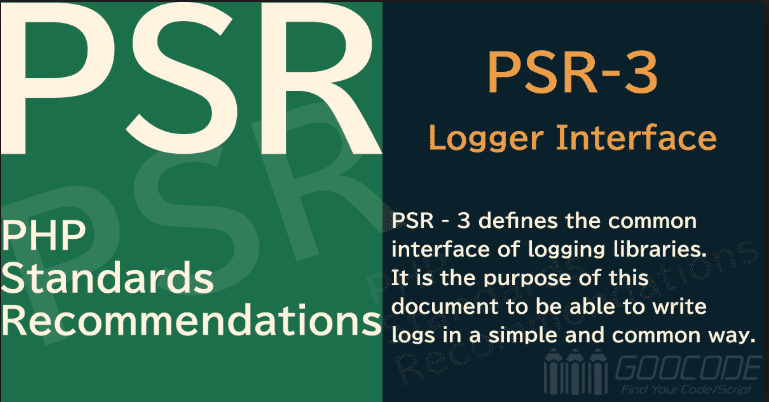
The implementers in this article refer to the class libraries or frameworks that implement the LoggerInterface interface. Conversely, they are the users of LoggerInterface.
1 Specification description
1.1 Basic specifications
The LoggerInterface interface defines eight methods for logging eight levels of logs defined in RFC 5424: debug, info, notice, warning, error, critical, alert, and emergency.
The ninth method - log, the first parameter is the level of the record. This method can be called with a predefined level constant as a parameter and must have the same effect as calling the above eight methods directly. If the passed level constant parameter is not predefined, an exception of type Psr\Log\InvalidArgumentException must be thrown. In the case of uncertainty, the user should not call this method with an unsupported level constant.
1.2 Recording information
Each of the above methods accepts a string type or an object with the __toString() method as the record information parameter, so that the implementor can treat it as a string, otherwise the implementer must convert it to a string. .
The record information parameter can carry a placeholder, and the implementer can replace the other with the corresponding value according to the context.
The placeholder must match the key name in the context array.
The name of the placeholder must be enclosed by a left curly brace { and a right parenthesis }. However, there must be no spaces between the curly braces and the names.
The name of the placeholder should consist only of A-Z, a-z, 0-9, underscore _, and English period. Other characters are reserved for future placeholder specifications.
Implementers can generate final logs by using different escaping and conversion strategies for placeholders.
The user should not escape the placeholder in advance without knowing the context.
The following is an example of a placeholder usage:
<php
* Replace placeholders in record information with context information
*/
Function interpolate($message, array $context = array())
{
/ / Build a replacement array of the key names contained in curly braces
$replace = array();
Foreach ($context as $key => $val) {
$replace['{' . $key . '}'] = $val;
}
// Replace the placeholder in the record information and finally return the modified record information.
Return strtr($message, $replace);
}
// Contains record information with curly braces placeholders.
$message = "User {username} created";
// A context array with replacement information, the key name is the placeholder name, and the key value is the replacement value.
$context = array('username' => 'bolivar');
// output "Username bolivar created"
Echo interpolate($message, $context);
1.3 context
Each record function accepts a context array parameter that is used to load information that the string type cannot represent. It can load any information, so the implementer must ensure that the information it loads is handled correctly, and that the data it loads must not throw an exception or generate PHP errors, warnings, or reminders (error, warning, notice).
To pass an Exception object via a context parameter, you must use 'exception' as the key name.
It is common to record exception information, so if it can be implemented at the bottom of the record class library, it will enable the implementer to extract the exception information.
Of course, when using it, the implementor must ensure that the key with the key name 'exception' is really an Exception, after all, it can load any information.
1.4 helper classes and interfaces
The Psr\Log\AbstractLogger class makes it easy to implement the LoggerInterface interface by inheriting it and implementing the log method, while the other eight methods are able to pass record information and context information to it.
Similarly, using Psr\Log\LoggerTrait also requires implementing the log method. However, it's important to note that the implement LoggerInterface is required before the traits reusable code block can't implement the interface.
The Psr\Log\NullLogger interface provides the user with an alternate log "black hole" when no logger is available. However, logging with conditional checking may be a better approach when the construction of the context is very resource intensive.
The Psr\Log\LoggerAwareInterface interface includes only one
The setLogger(LoggerInterface $logger) method, which the framework can use to automatically connect to any logging instance.
The Psr\Log\LoggerAwareTrait trait reusable code block can be used in any class, and the equivalent interface can be easily implemented with the $this->logger provided by it.
The Psr\Log\LogLevel class loads eight record level constants.
2 bags
The above interfaces, classes and related exception classes, as well as a series of implementation detection files, are included in the psr/log package.
3. Psr\Log\LoggerInterface
<?php
Namespace Psr\Log;
/**
* Log record instance
*
* Log information variable -- message, ** must be a string or an object that implements the __toString() method.
*
* The log information variable ** can contain a placeholder such as "{foo}" (for foo).
* It will be replaced by the key value in the context array with the key name "foo".
*
* A context array can carry arbitrary data. The only restriction is that when it carries an exception object, its key name must be "exception".
*
* For details, please refer to: https://github.com/PizzaLiu/PHP-FIG/blob/master/PSR-3-logger-interface-cn.md
*/
Interface LoggerInterface
{
/**
* System is not available
*
* @param string $message
* @param array $context
* @return null
*/
Public function emergency($message, array $context = array());
/**
* **Must ** Take action immediately
*
* For example: If the entire site is broken, the database is unavailable, or other circumstances, ** should ** send an alert message to wake you up.
*
* @param string $message
* @param array $context
* @return null
*/
Public function alert($message, array $context = array());
/**
* Emergency situations
*
* For example: Program components are not available or unexpected exceptions occur.
*
* @param string $message
* @param array $context
* @return null
*/
Public function critical($message, array $context = array());
/**
* Errors that occur during runtime, do not require immediate action, but must be documented for testing.
*
* @param string $message
* @param array $context
* @return null
*/
Public function error($message, array $context = array());
/**
* A non-erroneous exception occurred.
*
* For example: using a deprecated API, using an API incorrectly or an unintended unnecessary error.
*
* @param string $message
* @param array $context
* @return null
*/
Public function warning($message, array $context = array());
/**
* General important events.
*
* @param string $message
* @param array $context
* @return null
*/
Public function notice($message, array $context = array());
/**
* important events
*
* For example: user login and SQL record.
*
* @param string $message
* @param array $context
* @return null
*/
Public function info($message, array $context = array());
/**
*debug details
*
* @param string $message
* @param array $context
* @return null
*/
Public function debug($message, array $context = array());
/**
* Any level of logging
*
* @param mixed $level
* @param string $message
* @param array $context
* @return null
*/
Public function log($level, $message, array $context = array());
}
4. Psr\Log\LoggerAwareInterface
<?php
Namespace Psr\Log;
/**
* logger-aware definition instance
*/
Interface LoggerAwareInterface
{
/**
* Set up a logging instance
*
* @param LoggerInterface $logger
* @return null
*/
Public function setLogger(LoggerInterface $logger);
}
5. Psr\Log\LogLevel
<?php
Namespace Psr\Log;
/**
* Log level constant definition
*/
Class LogLevel
{
Const EMERGENCY = 'emergency';
Const ALERT = 'alert';
Const CRITICAL = 'critical';
Const ERROR = 'error';
Const WARNING = 'warning';
Const NOTICE = 'notice';
Const INFO = 'info';
Const DEBUG = 'debug';
}