PHP PSR-1 basic code specification
- 2-02-2019, 17:06
- 1516
Encoding specifications and standards are extremely important when working with groups to develop PHP projects. Next, let's revisit the PHP code related specifications developed by the FIG organization. The current general specifications are: PSR-1 basic code specification, PSR-2 code style specification, PSR-3 log interface specification, PSR-4 Autoloader automatic loading specification.
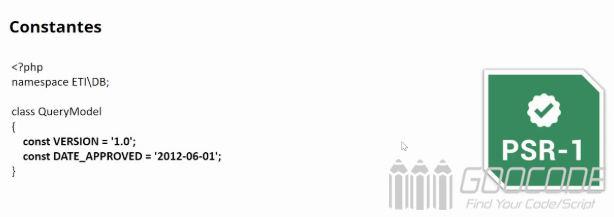
The PSR-1 Basic Code Specification establishes standards for the basic elements of the code to ensure a high degree of interoperability between shared PHP code.
Keywords "MUST", "MUST NOT", "REQUIRED", "SHALL", "No" (" SHALL NOT"), "SHOULD", "SHOULD NOT", "RECOMMENDED", "MAY", and "OPTIONAL" A detailed description of ") can be found in RFC 2119.
1. Overview
PHP code files must start with a <?php or <?= tag;
PHP code files must be encoded in UTF-8 without BOM;
PHP code should only define declarations such as classes, functions, constants, etc., or other operations that produce dependent effects (such as generating file output and modifying .ini configuration files, etc.), which can only be selected.
The namespace and class must conform to the PSR's autoloading specification: one of PSR-0 or PSR-4;
Class naming must follow the hump naming convention beginning with StudlyCaps capitalization;
Constants in a class All letters must be capitalized, with words separated by underscores;
The method name must conform to the camelCase-style lowercase opening hump naming convention.
2. File
2.1. PHP tags
PHP code must use a long tag or a <?= ?> short output tag;
Never use other custom labels.
2.2. Character encoding
PHP code must and can only use UTF-8 encoding without BOM.
2.3. Subordinate effects (side effects)
A PHP file should either define new declarations, such as classes, functions, or constants that do not produce subordinate effects, or only logical operations that produce subordinate effects, but not both.
The term "side effects" means the logical operation performed only by including files, not directly declaring classes, functions, constants, etc.
"Dependent effects" include but are not limited to: generating output, direct require or include, connecting to external services, modifying ini configuration, throwing errors or exceptions, modifying global or static variables, reading or writing files, and so on.
The following is a counterexample, a code that contains a statement and produces a dependent effect:
Here's an example of a code that only contains declarations that don't produce subordinate effects:
3. Namespaces and classes
The namespace and the naming of the class must follow PSR-0. (PSR-0 is deprecated)
According to the specification, each class is a single file, and the namespace has at least one level: the top-level organization name (vendor name).
Class naming must follow the hump naming convention beginning with StudlyCaps capitalization.
PHP 5.3 and later versions of the code must use a formal namespace.
E.g:
Versions 5.2.x and earlier should use the pseudo-namespace convention, which is predicated to use the top-level vendor name (vendor_) as the class prefix.
4. Constants, properties, and methods of a class
"Class" here refers to all classes, interfaces, and reusable traits (traits)
4.1. Constants
All letters in a class's constant must be capitalized, separated by an underscore.
Refer to the following code:
4.2. Properties
The property name of the class can follow the hump-style ($StudlyCaps) at the beginning of the capital, the camel ($camelCase) at the beginning of the lowercase, or the underscore ($under_score). This specification is not mandatory, but no matter which naming method is followed. , should be consistent within a certain range. This range can be the entire team, the entire package, the entire class, or the entire method.
4.3. Method
The method name must conform to the camelCase() lowercase beginning hump naming convention.
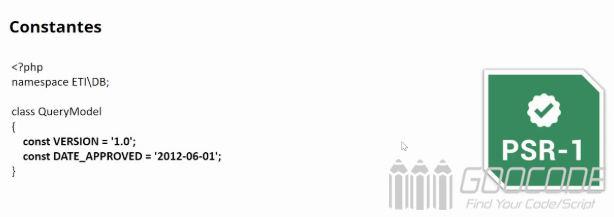
The PSR-1 Basic Code Specification establishes standards for the basic elements of the code to ensure a high degree of interoperability between shared PHP code.
Keywords "MUST", "MUST NOT", "REQUIRED", "SHALL", "No" (" SHALL NOT"), "SHOULD", "SHOULD NOT", "RECOMMENDED", "MAY", and "OPTIONAL" A detailed description of ") can be found in RFC 2119.
1. Overview
PHP code files must start with a <?php or <?= tag;
PHP code files must be encoded in UTF-8 without BOM;
PHP code should only define declarations such as classes, functions, constants, etc., or other operations that produce dependent effects (such as generating file output and modifying .ini configuration files, etc.), which can only be selected.
The namespace and class must conform to the PSR's autoloading specification: one of PSR-0 or PSR-4;
Class naming must follow the hump naming convention beginning with StudlyCaps capitalization;
Constants in a class All letters must be capitalized, with words separated by underscores;
The method name must conform to the camelCase-style lowercase opening hump naming convention.
2. File
2.1. PHP tags
PHP code must use a long tag or a <?= ?> short output tag;
Never use other custom labels.
2.2. Character encoding
PHP code must and can only use UTF-8 encoding without BOM.
2.3. Subordinate effects (side effects)
A PHP file should either define new declarations, such as classes, functions, or constants that do not produce subordinate effects, or only logical operations that produce subordinate effects, but not both.
The term "side effects" means the logical operation performed only by including files, not directly declaring classes, functions, constants, etc.
"Dependent effects" include but are not limited to: generating output, direct require or include, connecting to external services, modifying ini configuration, throwing errors or exceptions, modifying global or static variables, reading or writing files, and so on.
The following is a counterexample, a code that contains a statement and produces a dependent effect:
<?php
// Dependent effect: Modify the ini configuration
ini_set('error_reporting', E_ALL);
// Subordinate effect: introduction of files
include "file.php";
// Dependent effect: generate output
echo "\n";
// Declare function
function foo()
{
// Main body of the function
}
Here's an example of a code that only contains declarations that don't produce subordinate effects:
<?php
//Declare function
function foo()
{
// Main body of the function
}
// Conditional statement ** not ** belongs to subordinate effect
if (! function_exists('bar')) {
function bar()
{
// Main body of the function
}
}
3. Namespaces and classes
The namespace and the naming of the class must follow PSR-0. (PSR-0 is deprecated)
According to the specification, each class is a single file, and the namespace has at least one level: the top-level organization name (vendor name).
Class naming must follow the hump naming convention beginning with StudlyCaps capitalization.
PHP 5.3 and later versions of the code must use a formal namespace.
E.g:
<?php
// PHP 5.3 and later versions
namespace Vendor\Model;
class Foo
{
}
Versions 5.2.x and earlier should use the pseudo-namespace convention, which is predicated to use the top-level vendor name (vendor_) as the class prefix.
<?php
//5.2.x and previous versions
class Vendor_Model_Foo
{
}
4. Constants, properties, and methods of a class
"Class" here refers to all classes, interfaces, and reusable traits (traits)
4.1. Constants
All letters in a class's constant must be capitalized, separated by an underscore.
Refer to the following code:
<?php
namespace Vendor\Model;
class Foo
{
const VERSION = '1.0';
const DATE_APPROVED = '2012-06-01';
}
4.2. Properties
The property name of the class can follow the hump-style ($StudlyCaps) at the beginning of the capital, the camel ($camelCase) at the beginning of the lowercase, or the underscore ($under_score). This specification is not mandatory, but no matter which naming method is followed. , should be consistent within a certain range. This range can be the entire team, the entire package, the entire class, or the entire method.
4.3. Method
The method name must conform to the camelCase() lowercase beginning hump naming convention.
Comments (0)